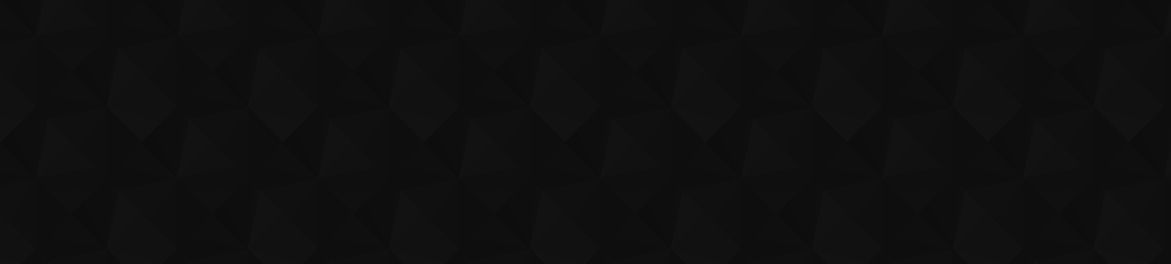
- 2 678
- 6 191 216
Cybrosys Technologies
India
Приєднався 13 чер 2009
We are Odoo Gold Partner standing par excellence in Odoo Implementation, Customization, and allied services. We do Source code sales. The latest research and developments in Cybrosys are being conducted in Blockchain. Our robust quality products and services are widely used by clients residing across in India, USA, UK, Middle East & Europe
Cybrosys Technologies is a private limited ISO certified company that bewitches with technology and software, that ensures delivering high-quality software products worldwide.
Our Odoo Services
1. Odoo Customization
2. Odoo Support & Maintenance
3. Odoo Implementation
4. Odoo Integration
5. Odoo Migration
Cybrosys provides tremendous scope for Odoo ERP implementation and further customizations upon request.
Website Link: www.cybrosys.com/
Cybrosys Technologies is a private limited ISO certified company that bewitches with technology and software, that ensures delivering high-quality software products worldwide.
Our Odoo Services
1. Odoo Customization
2. Odoo Support & Maintenance
3. Odoo Implementation
4. Odoo Integration
5. Odoo Migration
Cybrosys provides tremendous scope for Odoo ERP implementation and further customizations upon request.
Website Link: www.cybrosys.com/
Dictionaries in Python | EP-9 Python for Beginners Tutorials | Dictionaries Built-in Functions
"""Dictionaries are used to store data values in key:value pairs.
A dictionary is a collection which is ordered*, changeable and
do not allow duplicates.Dictionaries are written with curly brackets,
and have keys and values:
syntax:
dict_var = {
key1 : value1,
key2 : value2, …..
}
"""
#1.Empty dictionary
empty_dict ={
}
print("empty dictionary:",empty_dict)
#2.How to create dictionary
dict_1 ={
1:"cybrosys",
2:"technolgies"
}
print(dict_1)
#3.Different ways to create dictionary
dict_2=({1:"qwerty",2:"asdfg",3:"ghkjk"})
print(dict_2)
dict_3 = dict([(1,"a"),(2,"b")])
print(dict_3)
#4.getting length of dictionary
print(len(empty_dict))
print(len(dict_1))
print(len(dict_2))
#5.Dictionary items
"""syntax:
object.get(key)"""
data ={
1:"cybrosys",
2:"ground floor",
4:"Kinfra techno park"
}
print(data.get(1))
print(data.get(3))
x= data.get(4)
print(x)
#6.get key
"""syntax:
print(object.keys())"""
print(data.keys())
#7.get values
"""syntax:
print(object.values())"""
print(data.values())
#8.get items()
"""The items() method will return each item in a dictionary,
as tuples in a list.The returned list is a view of the items of
the dictionary, meaning that any changes done to the dictionary
will be reflected in the items list.
print(object.items())
where object is the data variable
"""
new = {
"a":1,
"b":2
}
print(new.items())
#9.Change Values of dictionary
(""" syntax:
object[key] = value """)
this_year={
"year":2018
}
this_year["year"]=2024
print(this_year)
#10.add dictionary
#1.update()
"""The update() method will update the dictionary with the items
from the given argument.The argument must be a dictionary,
or an iterable object with key:value pairs.
syntax:
object.update({key: value})
"""
abcd ={
"year":2021
}
abcd.update({"year":2025})
print(abcd)
#2.add
"""Adding an item to the dictionary is done by using a
new index key and assigning a value to it.
syntax:
object[key] = value
"""
abcd["color"] ="red"
print(abcd)
#11.remove dictionary items
#1.pop()
"""The pop() method removes the item with the specified key name
syntax:object.pop("key")
"""
abcd.pop("color")
print(abcd)
#2.popitem()
"""The popitem() method removes the last inserted item
(in versions before 3.7, a random item is removed instead).
syntax:
object.popitem()"""
b={
1:"q",
2:"h"
}
b.popitem()
print(b)
#3.del()
"""The del keyword removes the item with the specified key name
del object[key]"""
c={
4:"q",
5:"r"
}
del c[4]
print(c)
#4.clear()
"""The clear() method empties the dictionary
object.clear()
"""
c.clear()
print(c)
#Copy Dictionaries
"""copy keyword copies the item of one dictionary to
another dictionary
syntax:object.copy()
"""
ghk={
7:"t",
8:"k"
}
demo = ghk.copy()
print(demo)
#PythonForBeginners #PythonDictionaries #PythonBuiltInFunctions #LearnPython #CodingForBeginners #PythonProgramming #pythoncode #pythontips #keyvaluepairs #datastructures #efficientcoding #pythonhelp #pythoncommunity #pycom
Connect With Us:
-------------
➡️ Website: www.cybrosys.com/
➡️ Email: info@cybrosys.com
➡️ Twitter: cybrosys
➡️ LinkedIn: www.linkedin.com/company/cybrosys/
➡️ Facebook: cybrosystechnologies
➡️ Instagram: cybrosystech
➡️ Pinterest: pinterest.com/cybrosys/
A dictionary is a collection which is ordered*, changeable and
do not allow duplicates.Dictionaries are written with curly brackets,
and have keys and values:
syntax:
dict_var = {
key1 : value1,
key2 : value2, …..
}
"""
#1.Empty dictionary
empty_dict ={
}
print("empty dictionary:",empty_dict)
#2.How to create dictionary
dict_1 ={
1:"cybrosys",
2:"technolgies"
}
print(dict_1)
#3.Different ways to create dictionary
dict_2=({1:"qwerty",2:"asdfg",3:"ghkjk"})
print(dict_2)
dict_3 = dict([(1,"a"),(2,"b")])
print(dict_3)
#4.getting length of dictionary
print(len(empty_dict))
print(len(dict_1))
print(len(dict_2))
#5.Dictionary items
"""syntax:
object.get(key)"""
data ={
1:"cybrosys",
2:"ground floor",
4:"Kinfra techno park"
}
print(data.get(1))
print(data.get(3))
x= data.get(4)
print(x)
#6.get key
"""syntax:
print(object.keys())"""
print(data.keys())
#7.get values
"""syntax:
print(object.values())"""
print(data.values())
#8.get items()
"""The items() method will return each item in a dictionary,
as tuples in a list.The returned list is a view of the items of
the dictionary, meaning that any changes done to the dictionary
will be reflected in the items list.
print(object.items())
where object is the data variable
"""
new = {
"a":1,
"b":2
}
print(new.items())
#9.Change Values of dictionary
(""" syntax:
object[key] = value """)
this_year={
"year":2018
}
this_year["year"]=2024
print(this_year)
#10.add dictionary
#1.update()
"""The update() method will update the dictionary with the items
from the given argument.The argument must be a dictionary,
or an iterable object with key:value pairs.
syntax:
object.update({key: value})
"""
abcd ={
"year":2021
}
abcd.update({"year":2025})
print(abcd)
#2.add
"""Adding an item to the dictionary is done by using a
new index key and assigning a value to it.
syntax:
object[key] = value
"""
abcd["color"] ="red"
print(abcd)
#11.remove dictionary items
#1.pop()
"""The pop() method removes the item with the specified key name
syntax:object.pop("key")
"""
abcd.pop("color")
print(abcd)
#2.popitem()
"""The popitem() method removes the last inserted item
(in versions before 3.7, a random item is removed instead).
syntax:
object.popitem()"""
b={
1:"q",
2:"h"
}
b.popitem()
print(b)
#3.del()
"""The del keyword removes the item with the specified key name
del object[key]"""
c={
4:"q",
5:"r"
}
del c[4]
print(c)
#4.clear()
"""The clear() method empties the dictionary
object.clear()
"""
c.clear()
print(c)
#Copy Dictionaries
"""copy keyword copies the item of one dictionary to
another dictionary
syntax:object.copy()
"""
ghk={
7:"t",
8:"k"
}
demo = ghk.copy()
print(demo)
#PythonForBeginners #PythonDictionaries #PythonBuiltInFunctions #LearnPython #CodingForBeginners #PythonProgramming #pythoncode #pythontips #keyvaluepairs #datastructures #efficientcoding #pythonhelp #pythoncommunity #pycom
Connect With Us:
-------------
➡️ Website: www.cybrosys.com/
➡️ Email: info@cybrosys.com
➡️ Twitter: cybrosys
➡️ LinkedIn: www.linkedin.com/company/cybrosys/
➡️ Facebook: cybrosystechnologies
➡️ Instagram: cybrosystech
➡️ Pinterest: pinterest.com/cybrosys/
Переглядів: 96
Відео
How to Manage Bill of Material BOM in Odoo 17 Manufacturing App | Odoo 17 Functional Tutorials
Переглядів 8323 години тому
In Odoo, BOM stands for Bill of Materials. It's essentially a recipe or blueprint for a manufactured product or kit. It lists out all the individual components required, along with their exact quantities, to create the finished good. Odoo's BOM functionality goes beyond just a parts list, however. It can also include: Assembly instructions: You can include step-by-step instructions to guide wor...
Take User Input () in Python | EP-8 Python for Beginners | Input Function in Python | Python Videos
Переглядів 1012 години тому
Developers frequently have to communicate with users in order to obtain information or deliver a certain outcome. These days, the majority of programs solicit the user for input using a dialog box. input (): This function first takes the input from the user and converts it into a string. The type of the returned object always will be " class ‘str’ ". # Python program showing # a use of input() ...
How to Issue Credit Note in Odoo 17 Accounting | Issuing Credit Note | Odoo 17 Functional Tutorials
Переглядів 452 години тому
A credit note in Odoo is a document used to record a decrease in the amount a customer owes the business. There are a few common reasons why you might create a credit note in Odoo: A customer returns products: If a customer returns products that they previously purchased, you can create a credit note to reflect the fact that they now owe you less money. The customer overpays an invoice: If a cu...
What are Data Types in Python | EP-7 Python for Beginners | All Python Data Types | Python Tutorials
Переглядів 2084 години тому
# Datatypes Python Data types are the classification or categorization of data items. It represents the kind of value that tells what operations can be performed on a particular data. Since everything is an object in Python programming, Python data types are classes and variables are instances (objects) of these classes. The following are the standard or built-in data types in Python: Numeric S...
How to Configure Document From Email Alias in Odoo 17 Document App | Odoo 17 Functional Tutorials
Переглядів 634 години тому
Odoo, an open-source business management software, offers features to automate document generation based on emails. Here's a real-time scenario for a possible implementation: Scenario: Automatic Sales Order Confirmation via Email Customer Sends Email: A customer sends an email to the sales alias (e.g., [email address removed]) expressing their intent to purchase specific products. The email mig...
How to Swap Variables in Python | EP-6 Python for Beginners Tutorials | Swapping Variables in Python
Переглядів 1027 годин тому
Write a Python programme that switch the values of the two variables, x and y, provided. Let's examine many Python approaches for doing this task. method1: # Python program to demonstrate # swapping of two variables x = 10 y = 50 # Swapping of two variables # Using third variable temp = x x = y y = temp print("Value of x:", x) print("Value of y:", y) method 2:using comma operator # Python progr...
How to Automatically Send Customer Submissions in Odoo 17 Website App | Odoo 17 Functional Tutorials
Переглядів 707 годин тому
Odoo 17 website offers a built-in feature to automate sending customer submissions directly to your company email. This eliminates manual data entry and streamlines the process of receiving customer inquiries, feedback, or other forms. There are two main reasons why you might receive emails from an Odoo website form: Contact Form Submission: Odoo is a popular open-source business application su...
How to Find & Print Address of a Variable in Python | EP-5 Python for Beginners | Python Variables
Переглядів 19514 годин тому
How to find and print the address of the Python variable It can be done in these ways: Using id() function Using addressof() function Using hex() function Method 1: Find and Print Address of Variable using id() We can get an address using id() function, id() function gives the address of the particular object. Syntax: id(object) where, object is the data variables. list_ a = [1, 2, 3, 4, 5] pri...
Odoo 17 Project Management Webinar 2024 | Odoo 17 Project Management | Odoo 17 Functional Webinar
Переглядів 47214 годин тому
An organization's success depends on its projects. Every business has a unique collection of initiatives. Within a firm, there are a plethora of project kinds and approaches to managing them. A project goes through a number of steps from start to finish. 1. (00:00 - 1:42) - Webinar Introduction 2. (1:43 - 3:58) - Why Odoo ERP 3. (3:59 - 11:57) - Odoo 17 Project Features 4. (11:58 - 19:11) - Odo...
How to Change Shipping Label Size in Odoo 17 Inventory App | Odoo 17 Functional Tutorials
Переглядів 8516 годин тому
Before you purchase the label: Most shipping platforms allow you to choose the label size during the purchase process. Common sizes include A4 (for printing multiple labels on a sheet) and 4" x 6" (for individual labels). After you purchase the label: In general, you can't change the size of a shipping label after you've bought it. This is because carriers have specific requirements for label f...
What is Variables in Python | EP-4 Python for Beginners Tutorial | How to Create Variables in Python
Переглядів 15316 годин тому
In Python, a variable acts like a container that stores data values. These values can be anything from numbers and text to more complex data structures like lists or dictionaries. Here are some key points about variables in Python: Creating a Variable: Unlike some other programming languages, Python doesn't require you to declare a variable before using it. You create a variable simply by assig...
How to Create Project Task From Email Alias in Odoo 17 Project App | Odoo 17 Functional Tutorials
Переглядів 9916 годин тому
There are several advantages to creating project tasks from an email alias in Odoo, which can benefit both project managers and team members: Enhanced Workflow Efficiency: Reduced Manual Work: Eliminates the need to manually switch between email and Odoo to create tasks. Emails requesting work are automatically converted into tasks within the project, saving time and effort. Minimized Errors: A...
Getting Started With Python | EP-3 Python for Beginners | Free Python Tutorials | Free Python Videos
Переглядів 35919 годин тому
Learning Python is a great choice! Python is known for its easy-to-read syntax and versatility, making it an excellent language for beginners to delve into the world of coding. Here's a roadmap to get you started: 1. Installation: Head over to the cybrosys youtube ua-cam.com/video/1BeSnJ36SFs/v-deo.htmlsi=QX7L8hyWhURcrwtV to download and install Python. The installation process is straightforwa...
How to Create a Sequence Number in Odoo 17 | Odoo 17 Technical Videos | Odoo 17 Development Tutorial
Переглядів 7919 годин тому
In Odoo, a sequence number serves as a unique identifier for records within specific models, ensuring each entry is distinct and sequentially ordered. This tutorial explores the creation and customization of sequence numbers in Odoo 17, focusing on their application in sales orders, purchases, and invoices. Discover how to personalize sequences with options like prefixing, suffixing, and paddin...
How to Install Python Interpreter & PyCharm in Ubuntu | EP-2 Python Installation | Free Python Video
Переглядів 23321 годину тому
How to Install Python Interpreter & PyCharm in Ubuntu | EP-2 Python Installation | Free Python Video
How to Invoice Shipping Cost to Customer in Odoo 17 | Odoo 17 Functional Tutorials | Odoo 17 Videos
Переглядів 9021 годину тому
How to Invoice Shipping Cost to Customer in Odoo 17 | Odoo 17 Functional Tutorials | Odoo 17 Videos
What is Python? | EP-1 Python Tutorial for Beginners | Introduction to Python | Free Python Courses
Переглядів 546День тому
What is Python? | EP-1 Python Tutorial for Beginners | Introduction to Python | Free Python Courses
How to Create a Vendor in Odoo 17 Purchase App | Odoo 17 Purchase Tutorials | Odoo 17 Tutorials
Переглядів 164День тому
How to Create a Vendor in Odoo 17 Purchase App | Odoo 17 Purchase Tutorials | Odoo 17 Tutorials
How to Subscribe Newsletter From Odoo 17 Website App | Odoo 17 Functional Tutorials | Odoo 17 Videos
Переглядів 65День тому
How to Subscribe Newsletter From Odoo 17 Website App | Odoo 17 Functional Tutorials | Odoo 17 Videos
How to Create Task Through Odoo 17 Website Form | Odoo 17 Functional Tutorials | Odoo 17 Videos
Переглядів 73День тому
How to Create Task Through Odoo 17 Website Form | Odoo 17 Functional Tutorials | Odoo 17 Videos
How to Manage Employee Performance in Horilla HRMS | Free HR Software | Horilla Performance Software
Переглядів 9614 днів тому
How to Manage Employee Performance in Horilla HRMS | Free HR Software | Horilla Performance Software
How to Apply for a Job Through Odoo17 Website App | Odoo 17 Functional Tutorials
Переглядів 5614 днів тому
How to Apply for a Job Through Odoo17 Website App | Odoo 17 Functional Tutorials
How to Create Lead/Opportunity From Odoo 17 Website App | Odoo 17 Functional Tutorials
Переглядів 8114 днів тому
How to Create Lead/Opportunity From Odoo 17 Website App | Odoo 17 Functional Tutorials
How to Create Ticket From Odoo 17 Website Form | Odoo 17 Website App | Odoo 17 Functional Tutorials
Переглядів 8914 днів тому
How to Create Ticket From Odoo 17 Website Form | Odoo 17 Website App | Odoo 17 Functional Tutorials
How to Create a Customer Through the Odoo 17 Website Form | Odoo 17 Functional Tutorials
Переглядів 10714 днів тому
How to Create a Customer Through the Odoo 17 Website Form | Odoo 17 Functional Tutorials
How to Maximize Your Profit in Rental Industry | How to Configure Rental Analysis in Odoo 17 Rental
Переглядів 10014 днів тому
How to Maximize Your Profit in Rental Industry | How to Configure Rental Analysis in Odoo 17 Rental
How to Manage Employee Offboarding in Horilla HRMS | Free HR Software | Horilla Offboarding Software
Переглядів 8314 днів тому
How to Manage Employee Offboarding in Horilla HRMS | Free HR Software | Horilla Offboarding Software
How to Manage Reporting in Events of Odoo 17 Events App | Odoo 17 Functional Tutorials
Переглядів 14614 днів тому
How to Manage Reporting in Events of Odoo 17 Events App | Odoo 17 Functional Tutorials
How to Manage Scraping of Product in Odoo 17 Manufacturing App | Odoo 17 Functional Tutorials
Переглядів 15921 день тому
How to Manage Scraping of Product in Odoo 17 Manufacturing App | Odoo 17 Functional Tutorials
Hello
Why we use python?for what purpose?
what a nice and descriptive video. I have been struggling to understand this since my background is in tech not finance, thank you very much for the explanations. you are a good teacher. Keep it up ! Live demos, and creation of leads as you teach makes it easy to follow through, thats what sets you apart.
You’re just reading every feature one by one. Anyone can do this :) no technicality explained. It’s basic common sense
How you show products storage it doesn't appear while they both products types appear ( consumer and service) Varraint are check
Can you upload a resume into Odoo instead of entering the information?
What about using the import module button is that a viable option? I am using a docker image to host odoo and havent mounted my image so I cant add files via a IDE.
why the 'Program trigger', 'Use points on' fields not editable in program form?
How do you add an existing contact into the email marketing module into a contact lists without having to import or add it manually??
Can you make a video of how to create and automatic rfq. For retail store with 1000 of items
this is just a over view video create a video on payroll module. how to create salary structure. this video is trash you only explain things create a functional video
why don't you add this website page on the desc?
Thank you for detailed information, how we can make a portal where employees individually login and get their Pay slips monthly and announcements. Thanks
Muchas gracias Miss Cybrosys por lo excelente tutoriales
Muchas gracias guapa em ayudo....
does adding a new cashier automatically raise your price as an additional user?
good video , help me , i want invoice of page contain header and footer and the invoice in the middle, these gives me many pages ?
🔥🔥
Where buy the module?
Thank u mam what your name
can you help me how to add print button in list view so selected rows can print together
In case we set manual re-ordering rules for multiple products, do we have any option if we can generate a single purchase order if the vendor is same for all the products. pls confirm.
Malayali alle?
If the purchase price / quantity needs to be modified in the purchase order by Company B, is there a way to automatically update the sales order price / quantity in Company A?
Thanks
How can I effectively manage, identify and track assets without serial numbers or any unique id of the asset (like 100 keyboards in different branches) without physically labeling each asset because for me labeling 100 of keyboards manually is not optimum solution if such case repeat again. So how to handle this situation and ensuring accurate inventory and traceability across multiple branches?
Where I can see expected recurring amount on forecast ? Example: I have 100 usd for expected revenue and 20 usd for recurring plan 3months, it means after I set expected closing on CRM e.g. expected closing date is June 2024, I have 20 usd (expected recurring revenue) for each month July, August, September. But report forecast did not show amount of recurring revenue on the right month.
How if customer want return item but he doesn’t paid i mean by customer account method in odoo the amount still in the reconciliation window
I am watching to refresh my python knowledge, It is interesting to see that unpacking collection in python. i never learned before, i saw similar in JavaScript array destructuring, it's been new knowledge
what about leads from Meta Business Suite
Thank you
How to use it
Thanks a lot for your awesome channel
Beginners can adapt very well
Thank you cybrosys ❤❤❤❤❤
Great effort for beginners 😊
Hi
HI IF I NEED TO ADD CONDITION TO ADD A PRODUCT THAT ACCORDING TO PRODUCT TAGS , HOW I CAN DO IT ? THANKS
Please email your complete scenario to odoo@cybrosys.com
Very helpful, thanks for your videos!
Glad you like them!
Mam what about earnings by this..!!
Mam what about earning in this ..?!!
Good work, I'm an Odoo Functional guy. Would like to learn Python from Cybrosys.
how to connect one contact to many companies?
Hi Jareena, I'm an Odoo Functional Consultant and I want to learn Python to become technical as well. I would definitely like to learn Python from Cybrosys in a fast and fun way.
Pick me please
Sound Great
Thank u so much maam, but we request can u segregate the tutorial video accourdily modules wise if possible.
We will try our best. Also please check this link www.cybrosys.com/odoo/videos/
thanks for nice tutorial for help for us using odoo.
You are welcome
nice tutorial thank u so much for help
You are most welcome
Can we create another company as separte database
You'll need separate license.